Adding Simple Functions to GraphWin
We call a function of type void func(GraphWin& gw); simple.
The add_simple_call() operation of GraphWin
can be used to add (buttons
for starting) simple functions to an existing menu or the main menu
bar.
void gw.add_simple_call
(void (*func)(GraphWin&), string label, int menu_id=0);
adds a new button with label label to the menu with
menu id menu_id . Whenever this button is pressed in
edit mode func(gw) is called.
|
Example:
Assume we want to add a button to the main menu that runs a DFS
algorithm of type
void dfs(graph& G, node s,
node_array<bool> reached);
on the current graph. We write
a simple function void run_dfs(GraphWin&) that tells
GraphWin how to call DFS and how to display the result.
On the right there is a screenshot of the program. Clicking on
the picture shows the window in original
size.
|
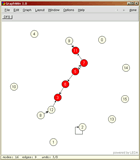 |
#include <LEDA/graphics/graphwin.h>
#include <LEDA/graph/graph.h>
#include <LEDA/graph/node_array.h>
#include <LEDA/graph/graph_alg.h>
using namespace leda;
void run_dfs(GraphWin& gw)
{
//provide arguments
graph& G=gw.get_graph();
node s=gw.ask_node();
node_array<bool> reached(G,false);
DFS(G,s,reached); //call function
//display result
node v; forall_nodes(v,G)
if (reached[v]) gw.set_color(v,red);
}
int main()
{
GraphWin gw;
gw.add_simple_call(run_dfs,"DFS");
gw.display(); gw.edit();
gw.get_window().screenshot("gw_simple_functions");
return 0;
}
|
|
See also:
Customizing the Interactive Interface
GraphWin
Interactive Interface
Adding New Menus
Adding GraphWin Member Functions
Adding Families of Functions
Complete Example for Customizing
the Interface
Windows and Panels
Panel Buttons
Graph Data Types
Node Arrays
Depth First Search
and Breadth First Search
Manual Pages:
Manual
Page GraphWin
|