Example Minkowski Sums
The following program generates two simple polygons P1
and P2 and computes the Minkowski sum GPSUM and the
Minkowski difference GPDIFF .
Then it draws P1 in red and P2 in green
GPSUM in black and GPDIFF in blue.
On the right there is a screenshot of the program. Clicking on
the picture shows the window
in original size.
|
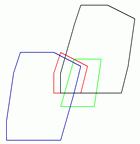 |
#include <LEDA/core/list.h>
#include <LEDA/geo/rat_point.h>
#include <LEDA/geo/rat_segment.h>
#include <LEDA/geo/rat_gen_polygon.h>
#include <LEDA/graphics/window.h>
#include <LEDA/geo/geo_alg.h>
using namespace leda;
int main()
{
list<rat_point> L1;
L1.push(rat_point(0,10)) ;L1.push(rat_point(0,25));
L1.push(rat_point(5,40)); L1.push(rat_point(25,30));
L1.push(rat_point(20,10));
rat_polygon P1(L1);
list<rat_point> L2;
L2.push(rat_point(5,0)); L2.push(rat_point(15,35));
L2.push(rat_point(35,35)); L2.push(rat_point(30,0));
rat_polygon P2(L2);
rat_gen_polygon GPSUM=MINKOWSKI_SUM(P1,P2);
rat_gen_polygon GPDIFF=MINKOWSKI_DIFF(P1,P2);
window W;
W.init(-45,85,-45);
W.open(); W.display();
W.set_line_width(2);
W.draw_polygon(P1.to_polygon(),red);
W.draw_polygon(P2.to_polygon(),green);
W.read_mouse();
W.draw_polygon(GPSUM.to_gen_polygon());
W.read_mouse();
W.draw_polygon(GPDIFF.to_gen_polygon(),blue);
W.read_mouse();
W.screenshot("minkowski_sum");
return 0;
}
|
See also:
Minkowski Sums
Data Types for 2-D Geometry
Windows and Panels
Linear Lists
Geometry Algorithms
Geometry
Graphs and Related Data Types
GeoWin
Number Types
Manual Entries
Manual
Page of Geometry Algorithms
|