Example Line Segment Intersections
The following program shows how to compute the intersections between
50 random segments. After computing the intersection, a window
is used to display the segments and the intersections.
On the right you see a set of 50 segments in the plane, the intesections
are drawn in red. The picture is a screenshot from the program below.
Remark: LEDA provides several variants for computing intersections
between line segments using different algorithms and satisfying
different output requirements. See the Manual
Page of Geometry Algorithms and the LEDA
Book for details.
|
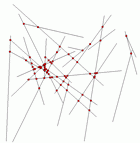 |
#include <LEDA/core/list.h>
#include <LEDA/geo/point.h>
#include <LEDA/geo/random_point.h>
#include <LEDA/graphics/window.h>
#include <LEDA/geo/geo_alg.h>
using namespace leda;
int main()
{
list<point> LP;
random_points_in_square(50,100,LP);
list<segment> L;
while (!LP.empty()) {
point p=LP.pop();point q=LP.pop();
L.append(segment(p,q));
}
list<point> intersections;
SEGMENT_INTERSECTION(L,intersections);
window W;
W.init(-110,110,-110);
W.open(); W.display();
W.set_node_width(3);
segment s; forall(s,L) W.draw_segment(s);
W.read_mouse();
point p; forall(p,intersections) W.draw_filled_node(p,red);
W.read_mouse();
W.screenshot("segment_intersection");
return 0;
}
|
See also:
Data Types for 2-D Geometry
Linear Lists
Windows and Panels
Writing Kernel Independent Code
Geometry Algorithms
Geometry
GeoWin
Number Types
Manual Entries
Manual
Page of Geometry Algorithms
|